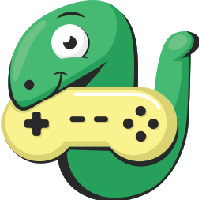
Introduction
In this article, I will show you how to create simple 2-dimensional map based on Simplex Noise. We are going to write a map generator in Python using The Python Arcade as a game engine and OpenSimplex library as a noise generator. Let's go!
Prerequisites
- Basic knowledge about python.
- Basic knowledge about game engines.
What is this noise about?
I don't want to make a math science here. Let's keep things simple here.
We want our terrain:
- to make it looks like a real terrain
- to make it repetitive while using same seed
- to make it unique while using different seeds
Basic concept looks like this: there are known coordinates like X and Y and random constant called seed. We want to obtain Z coordinate (height) for each (X, Y) point. The magic is that with same seed there will be always same result. In my example I generate 2D map, but there is no obstacles to make it 3D. I created ten 16x16 sprites, each for different representation of Z coordinate. Z is going to have values from -1.0 to 1.0. Everything below 0.1 is going to be ocean, then I made some greenery, rocks and snow on top of mountains.
Preview:
Code review
Let me explain best parts:
Why reinvent the wheel? We are going to use opensimplex library which gives freedom in creation of Simplex noise. In this example I use uuid to generate a truly random grain. Let's move on to main methods:
return
=
=
return
Random seed generation is optional. In this example we are going to view maps generated by different seeds.
# Set the background color
# Sprite lists
=
=
=
=
=
=
=
=
=
=
=
=
=
=
=
=
=
In each update:
- arcade.SpriteList() with 16x16 sprites is cleared
- seed is randomly generated
- for each X and Y on the screen Z dimension is calculated
- depending on Z (height), sprite (water/ grass/rock/snow) is selected
- sprites are added to the arcade.SpriteList()
- back to point 1.
Preview
Code with sprites is avaivable on my github here.
TO-DO: It would be cool to also have an example where seed is static and we only move on single axis.